Including a custom font in my Rails app was trickier than I expected it to be. Specifically, I wanted to include Entypo so that I could use its fun social icons. The Entypo website provides a downloadable zipfile of individual svg files, which isn't what I wanted. I was eventually able to find, via the Google machine, the set of eot, svg, ttf, woff and css files that are generally needed in order to require the font in your app. In Rails 4, copying the 4 font files directly into your assets/fonts folder should automatically require them in your app. I placed my CSS file into the stylesheets folder and refreshed my app. I had added an icon to a view, and in its place was appearing a small rectangle. So the view knew to look for an icon, but it wasn't finding the character. Something about the way I had copied in the font files was not correct.
In your font's CSS file, you'll need to specify the file path to the eot/svg/ttf/woff font files. The default will look something like this:
@font-face {
font-family: 'entypo';
src: url('/assets/entypo.eot?71205724');
src: url('/assets/entypo.eot?71205724#iefix') format('embedded-opentype'),
url('/assets/entypo.woff?71205724') format('woff'),
url('/assets/entypo.ttf?71205724') format('truetype'),
url('/assets/entypo.svg?71205724#entypo') format('svg');
font-weight: normal;
font-style: normal;
}
Since the characters weren't loading, I figured there was something up with the relative path for each of these files. I played with the path for some time and wasn't able to find the combination of folders that would get the files to load. While researching alternatives to abstract out the literal paths of the files, I discovered the rails helper asset_path. It's used via ERB, like so:
@font-face {
font-family: 'entypo';
src:url(<%= asset_path 'entypo.eot?-pco6za' %>);
src:url(<%= asset_path 'entypo.eot?#iefix-pco6za' %>) format('embedded-opentype'),
url(<%= asset_path 'entypo.woff?-pco6za' %>) format('woff'),
url(<%= asset_path 'entypo.ttf?-pco6za' %>) format('truetype'),
url(<%= asset_path 'entypo.svg?-pco6za#entypo' %>) format('svg');
font-weight: normal;
font-style: normal;
}
Essentially this helper "computes the path to an asset in a public directory" (per Rails documentation). Using this helper will also allow any file references within these files to be processed by the Asset Pipeline. NOTE: It's imperative that you also change the file extension of the css file to .css.erb, otherwise your stylesheet will not be processed.
A few choice words on IcoMoon
I watched a great CSS-Tricks screencast called Creating and Using a Custom Icon Font. To quote this reference, "To be the most efficient with your icon font, you should only load the characters that you need to load. And to be flexible, it's nice to be able to add to a single icon font from multiple sets or from any vector/SVG anywhere at all. The IcoMoon app lets you do all of this from it's super simple interface. You choose the icons you want, map them to whatever characters you want, then export the HTML/CSS/Web Fonts in a ready to use format."
I found IcoMoon to be extremely easy to use and to require in my app, so I thought I'd share it with you all. Enjoy :)
READ THIS POST IF: you are deploying an app to Heroku and the build fails while compiling assets.
Scenario: I'm working on an app that is styled using a theme from a vendor. I've copied the CSS files I need into vendor/assets/stylesheets and the javascripts into vendor/assets/javascripts, without any nesting. All files seem to be properly required in my app; my manifests require the vendor/assets trees and the styling and behavior in my app are as I want them to be.
It comes time to push up to Heroku. git push heroku master
. She starts writing temp files, ok good... she runs rake assets:precompile, it's looking normal... and then precompiling assets fails. What gives?
All the things I tried: (skip to the fix)
Precompiling Assets
It seems reasonable to try to precompile assets before pushing up to Heroku and then running the build again. So I run rake assets:precompile locally and it passes without fail. I push up to Heroku, and the build works! But I notice that my javascripts are broken, both locally and remotely. There's a sidebar that should expand and collapse upon click of the menu icon, and it isn't working. Huh.
Mixed Dependencies
So I look into my javascripts manifest and I think jquery might be required twice. It feels wrong, but I remove //require jquery and //require jquery_ujs. And javascripts go back to normal! Ok, cool.
I continue business as usual. I decide to work on my login page. I click sign out and... what? Why am I served a rails error? It seems as though clicking the link that directs to users/sign_out is trying to take me to the users#show route, users/:id, because it thinks "sign_out" is a user_id. Devise was the first feature I implemented, over a week ago, and it's worked up til now. Weird. I troubleshoot this as best I know.
Route configuration
I change the order of the "devise_for" and "resources :users" in routes.rb. That doesn't do it. I remove "resources :users" completely. No dice.
The sign out route is inherited via Devise as sessions/users/sign_out, so it's tucked away in the Devise gem within the sessions controller and isn't actually present in my project. But I can run rails generate devise:controllers users
to generate this controller (and all devise controllers). I explicitly set devise_for :users, controllers: { sessions: "users/sessions" }
hoping that by explicitly telling my routes.rb file where the sessions routes lives, it will no longer confuse the sign out and show routes.
This doesn't do anything at all. I revert.
Asset loading
There's an entypo icon inside of my Sign Out link. Maybe having an asset inside of that link is breaking the link action? I remove it. No change. Revert.
Baseline compatibility: Does Devise play nice with Heroku?
How are my assets, devise, and heroku even related? Well, I start researching Devise and Heroku. Many sources confirm that sometimes compiling assets during a heroku push will break as a result of using Devise, but upon further research, this issue is specific to rails 3 and produces an error message dissimilar to that I was getting. So I ruled out Devise as the cause of the Heroku compile issue, and deduced that //require jquery and //require jquery_ujs had to be put back in.
Application configuration
I start vigorously searching stackoverflow.
I add <%= javascript_include_tag :defaults %> to application.html.erb. No change.
Javascript Manifest File (mounting order)
At this point, it seems like I need to diagnose why precompiling assets is break my javascripts in the first place. Are my scripts required in an incorrect order in the manifest file?
I use rake assets:clobber to remove the precompiled assets I had just generated (which deletes the public/assets folder).
I search the theme FAQs on the vendor site, but although the help topics are fairly thorough I find nothing referencing the mounting order of the javascript files.
I un-require the vendor tree and look back into my theme and decide to manually list out each vendor javascript that I need, in the exact same order as the theme's example files. Re-precompile. No change.
CSS
I need to look at this from a new angle. If precompiling is working locally, albeit breaking my javascript, why does compiling break in Heroku push? I look back at the error.
rake aborted!
Invalid CSS after
.....
Wait, invalid CSS? What is that about? When I precompile, only the javascript is broken.
THE FIX!!!!
I grab a brilliant Flatiron instuctor who suggests the issue might have something to do with my manifest file being a SASS document while the rest of my stylesheets are a combination of CSS and SASS. I deduce it might be a good idea to standardize to either all SASS or all CSS. I'm not even using any SASS, so I change all of the .scss file extentions to .css, including the manifest file, remove 'gem SASS-RAILS' from my Gemfile, bundle, commit, and push. It goes through. Heart racing. Open the heroku app. Styling is all in place. Sign in. Click the sidebar, it expands and collapses. Sign out.TA DA!!!
Oh my god. I can't believe I have spent 8 hours on this issue, and it was CSS the whole time - it had nothing to do with JQuery, or Devise, WHAT A RABBIT HOLE THAT WAS.
Moral of the story: If you try to push up to Heroku and your build is aborted in the middle of compiling assets, and you see an "invalid CSS" error message, check your file extensions FIRST.
Your issue is unlikely to be due to any of the following:
- Devise (specifically, the order of your routes in routes.rb probably doesn't matter, and whether your controllers are tucked into Devise or present in your app file tree shouldn't either.)
- Icons included in the display value of links
- The order of your files in the javascript manifest
- The presence of //require jquery and //require jquery_ujs in the javascript manifest. READ: DO NOT REMOVE THESE.
- Javascripts included manually in the head of application.html.erb
- The presence of old compiled assets
By the way, I couldn't find this information anywhere on stackoverflow. Watch me spread the gospel of this fix all over the internet.
Needless to say I'll be making a sacrifice to the Heroku gods tonight. Godspeed, dear Heroku users.
Rails generators are pure magic: we use them to create the basic framework for our rails apps so that we don't have to undergo the tedious exercise of creating the structure ourselves. There are 5 basic commands we use quite frequently that fall at various levels of abstraction. We choose to use one over the other depending on the level of complexity our models require. Although there are plenty of resources online that document rails generators and how to use them, I I couldn't find a single consolidated cheat sheet anywhere on the web. I've created a chart below that has already mitigated some of the confusion for me, so I wanted to share it with you, dear reader.
In order of abstraction from least to greatest the commands rank as follows:
- rails generate migration
- rails generate model
- rails generate controller
- rails generate resource
- rails generate scaffold
And the table below documents what you can expect to be created for you when you use each one:
Command |
Syntax: Model name + ? |
Model |
Migration |
Controller |
Views |
Test Suite |
Assets (Javascripts, Stylesheets) |
Route in config file |
Helper |
Migration |
attributes as name:type |
N |
Y |
N |
N |
N |
N |
N |
N |
Model |
attributes as name:type |
Y |
Y |
N |
N |
model |
N |
N |
N |
Controller |
views |
N |
N |
Y |
Y |
controller |
Y |
Y |
Y |
Resource |
attributes as name:type |
Y |
Y |
Y |
folder; no files |
controller, model |
Y |
N |
Y |
Scaffold |
attributes as name:type |
Y |
Y |
Y |
Y |
controller, model |
Y |
Y |
Y |
Don't forget, if you ever choose the wrong one and want to undo, you can use the rails destroy command. For example, if you created a scaffold for Becca but realized you only needed a controller, you could execute:
rails destroy scaffold becca
rails generate controller becca index show
Just remember that it will remove any and all files related to your Becca model, so use with caution.
:)
Question: What is a slug?
Answer: This is a slug:

source: http://news.gardentoolbox.co.uk/articles/slugs-removing-them-naturally-and-un-naturally
A Second Answer: This is also a slug:

Which did you think I meant? And why is this relevant?
Now that I'm building web apps, it's important for me to be conscious of creating RESTful URLs. REST, which stands for Representational State Transfer, refers to a set of conventions within web architecture that define best practices or standards for representing resources on the web (where a resource is any data requested by the user).
The REST philosophy has several components, but a fundamental tenet is that URLs on the web should be readable and somewhat intuitive. In order to comply with the RESTful pattern, it is generally preferable for URLs to represent what the page displays in a human-readable format. If I were to send you a link to my Twitter page, it's pretty awesome that I can direct you to
where bshap27 is my Twitter handle, and it's as simple as that. Easy to understand, easy to remember. It would be pretty annoying if the URL were something nonsensical like
or even something like
Neither of those paths are RESTful, because I can't intuit what they mean. Now, what if I wanted to send you a link to a new song I recorded called "I Love Sunshine"? Spaces can't be used in a URL. This is where slugs come in.
A slug, in this context, refers to a string of words that is stripped of spaces and filled instead with punctuation that is more compatible with a URL, usually a dash. When most browsers identify spaces in a URL, they automatatically render those spaces to a default string. A quick Google search of my own name shows me that Google changed the space in my search terms to the string "%20":
https://www.google.com/webhp?sourceid=chrome-instant&ion=1&espv=2&ie=UTF-8#q=becca%20shapiro => becca%20shapiro
But that's messy. So when we're creating URLs of our own, we follow REST conventions and use a slug.
(Disclaimer: not a real website or song).
Aesthetics and usability aren't the only reasons we use slugs. I mean, how often do people actually type out URLs that are more than like, 20 characters? Slugs don't just help PEOPLE find a website, they help SEARCH ENGINES find a website. Including search keywords in your site URL can increase your page rank. And when link contents are easily discernable from the URL, users are better able to gauge whether the content is relevant to them and are more likely to click. Hasn't a friend ever sent you an email with no subject and just a link? And has it ever happened to you that the link URL was jumbled, and you weren't sure if it was spam? Readable URLs prevent this conundrum. And therefore, slugs are important from an SEO perspective.
Now, at some point, really long URLs are excessive. And super long URLs can actually hurt SEO rather than bolster it because too many keywords can be perceived as junk. So from comments on StackOverflow and several other blogs and forums, I've done the work for you and deduced that a reasonable standard seems to be 5 words or less. A common practice is to remove terms like 'the', 'and', 'or', etc. in order to keep word/character count manageable.
Finally, you may be wondering about the etymology of the word slug. I was too. Enjoy.
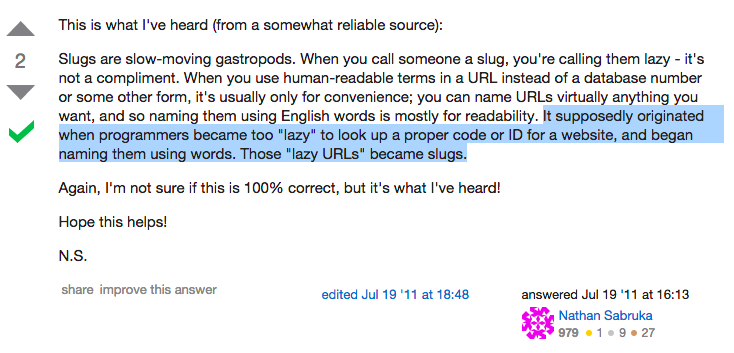
http://stackoverflow.com/questions/6750300/how-did-the-term-slug-as-in-urls-originate
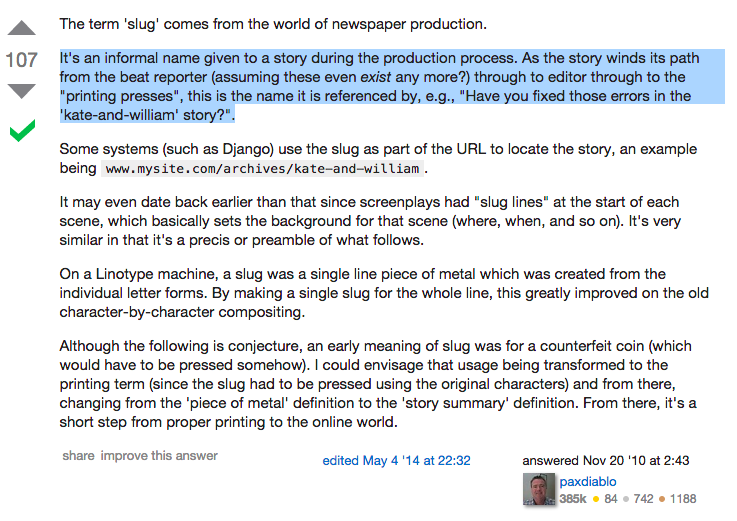
http://stackoverflow.com/questions/4230846/what-is-the-etymology-of-slug
Open Questions and Other Resources:
How would Roy Fielding, the inventor of REST, feel about bit.ly?
How about this? Google Researchers Introduce System To Rank Web Pages On Facts, Not Links
Further reading: SEO Beginners Guide to Permalinks and Slugs (note: look at the URL for this article. Very RESTful).
Did you know that if you lick a banana slug, your tongue goes numb? (Fun fact, I've done it).
Similar to the way avocados have ‘good fat’ so they aren’t bad for your health, Syntactic Sugar, in Ruby, is 'good sugar,' in that it makes Ruby delicious to write without compromising the integrity or specificity of your code (code health, if you will).
Yukihiro “Matz” Matsumoto created the Ruby language with beauty, art, and nature in mind, intending to equip the programmer with a linguistically intuitive language with which to build powerful, complex code. By abstracting frequently-occurring complex expressions to simpler commands, and offering built-in syntactic shortcuts, Ruby reads more like English than most other programming languages. “Syntactic sugar” refers to the linguistic flexibility deliberately built into the Ruby language that allows us to read and write functional code that’s easier on the eyes, requires fewer lines of code, and consequently induces fewer headaches. As a result, I imagine syntactic sugar is primarily what makes Ruby the most approachable language for programming newbies, not to mention the one most frequently taught in web development bootcamps.
Allow me to explain.
Syntactic Sugar Helps Code Read More Naturally
In Ruby, a method is always called on an object using dot notation. In the example below, the string "i am yelling" is my object and "upcase" is my method. Observe.
"i am yelling".upcase
=> "I AM YELLING"
You may be thinking, "But wait, what about methods like 'puts'? I don't use a dot there." And you'd be right. Because when you call the method puts, which prints a string to the screen, what you're really doing is calling the method on an implicit receiver, which is the object you are in - usually the default object, 'main'. Technically, when you write:
puts 'Becca is brilliant!'
what you're really telling the computer to do is
self.puts 'Becca is brilliant!'
If you're confused, you're in good company, and that's ok. I'll talk more about scope another time. Just take my word for it that writing 'self.puts' is the same as just writing 'puts,' and that syntactic sugar allows you to take this shortcut and eliminate the need for the dot.
This isn’t really any different than the way we approach the English language. We use syntactic sugar in everyday speech! Take contractions (won't, isn't, I'll, etc), for example. They make written and verbal speech sound more natural, or colloquial, to us.
For example, let's dissect this conversation which I may or may not have been subject to this weekend:
Best friend: “Why don’t you come to the party with me? Let’s just check it out for an hour. What do you mean you aren’t leaving the house? You’re never gonna find your sexy smart sensitive doctor prince charming on your couch! It’s you I’m looking out for!”
Now remove the contractions.
Best friend: “Why do not you come to the party with me? Let us just check it out for an hour. What do you mean you are not leaving the house? You are never going to find your sexy smart sensitive doctor prince charming on your couch! It is you I’m looking out for!”
Say it out loud. Aside from sounding snottier, it also just doesn’t roll off the tongue. Also, I know “gonna” isn’t a contraction, but you get my point.
Syntactic Sugar Helps Reduce Unnecessary Effort
Here's an array:
things_i_love = ['dark_chocolate', 'knitting', 'skydiving']
Let's say we wanted to see what the first item in my things_i_love
array is. We refer to items in arrays by their index, which are numbered starting at 0. So, to see what the first item is in this array, I could generally write:
things_i_love[0]
=> "dark_chocolate"
and that would return me the string "dark_chocolate." But I told you before that all methods call on an object using a dot. Where's the dot?
Well, the notation above is actually an abbreviated version of this:
things_i_love.[](0)
=> "dark_chocolate"
It looks gross without the sugar coat, right? What is this, even? Well, we're calling the method []
(which, out loud, would be "bracket") on the object things_i_love
, and passing the index of 0 to it as an argument. But that's so much more confusing than the first way. And because our friend Matz was concerned with elegance, he's constructed the language such that we can break syntactic rules in the interest of ease and clarity.
Now for an English comparison. I don’t know about you, but I hate typing on an iPhone. Now, this is kind of a bad example because sometimes autocomplete does exactly the opposite of what you want it to do.
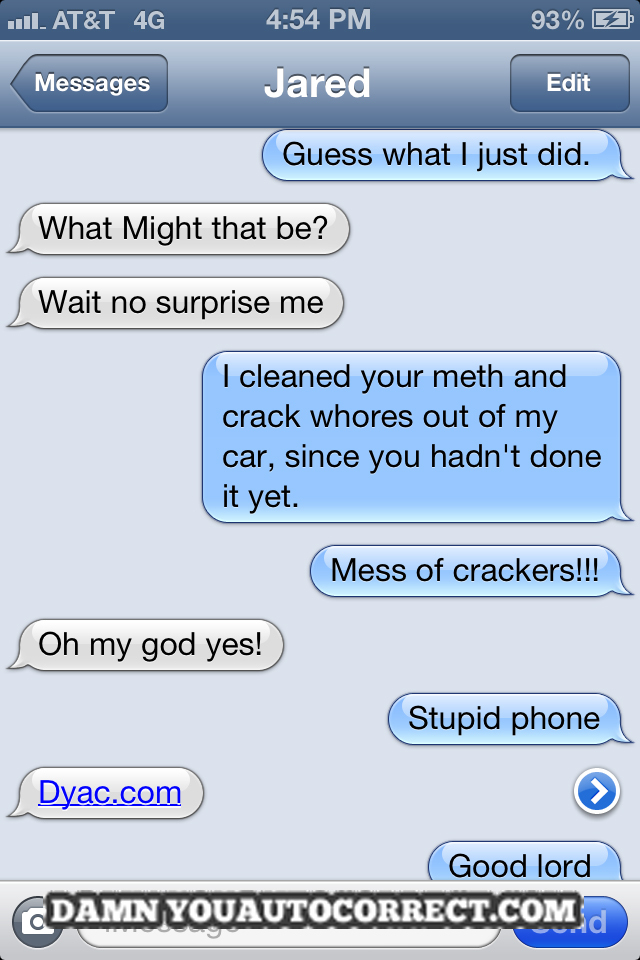
But it also allows me to type the letter ‘u’ and it will automatically convert it to the word ‘you’ so that I don’t sound like a pre-teen. This eliminates 2 letter-taps of effort and yields the same end result (a text message that most likely contains the dancing cat girls emoji).
Another thing humans do along these lines: we say "YOLO!" instead of always having to say “you only live once,” which of course you have to say all the time.
This is no different than taking advantage of syntactic sugar in Ruby to skirt the rules of conventional syntax.
Syntactic Sugar Helps Reduce Unnecessary Complexity
Let's say we have an array and we want to 'puts' out each item in the array.
things_i_love = ['dark_chocolate', 'knitting', 'skydiving']
To iterate over this array, I'd need to set up a loop that puts out each item, and in each cycle of the loop, increases the index by 1.
def puts_each_item array
index = 0 # starts my index at 0, sets up a counter
while index < array.length # sets up a loop that ends the program
# once the index matches the number of
# items in my array
puts array[index] # puts out the item for the current index
index += 1 # increments the index by 1
end # ends the loop
end # ends the program.
This is so annoying! I have to do that EVERY TIME I want to do something to each item in an array?
Nope, no I don't. In programming, we iterate over an array all the time. Ruby has iterator methods built in to make this easier for us. I could have taken this up a level of abstraction and simply written:
def puts_each_item array
array.each do |item| # calls the 'each' method, a built-in iterator,
# on my array object and specifies the
# variable name, in |bars|, to represent
# each item in the array.
puts item # compare this line to puts array[index], above
end # end loop
end # end program.
So much cleaner.
Now for an English example. I like to conjure an image of a micro-managing mom:
Mom: “Sweetie, could you help me prepare for dinner by putting 5 plates out? Make sure to fold a napkin and put it on the lefthand side of each plate. Then put a fork on top of the napkin and a knife to the right of the plate, and don’t forget 5 glasses!”
Mom could have just said, “Sweetie, could you set the table?” I know where everything goes. I’m 26. Thanks, Mom.
And so does Ruby. Although computers don’t have the power to make any assumptions on our behalf, in many cases we don’t need to take the computer through every step one by one, thanks to syntactic sugar. We can add a level or two of abstraction and save ourselves the time of dictating lengthy instructions.
......
So, do you see what I’m saying? We can consolidate words and syntax in our code, just like in written and spoken language, to remove clutter and thereby make our code easier to write and understand.
Syntactic Vinegar
I’d be remiss not to mention the antithesis to syntactic sugar: syntactic vinegar.
Syntactic vinegar is the exact opposite of syntactic sugar in that certain expressions in Ruby are deliberately ugly and/or complicated so as to discourage their use.
It amuses me to liken syntactic vinegar to the use of business jargon. Jargon exists in order for you to express yourself clearly within a certain context, but it tends to be exceptionally annoying outside of that context. For example, in an office setting you might need to say to your boss, “I’m sorry, I just don’t have the bandwidth to do that right now.” It’s probably the most professional way to express that you’re overwhelmed by your workload. However, if you were say that to your friends as an excuse not to go to a party, you’d just sound like an ass.
In Ruby, there’s a method called instance_variable_get
that allows you to find an instance variable given an instance of a class. To do this in a manner considered acceptable by most Rubyists, you'd make an attribute reader method at the time that the variable is defined, so that you can access the variable outside of the class. The instance_variable_get
method is a comparatively ‘dirty’ way to access that same information. A wise instructor at Flatiron School wrote:
I believe that instance_variable_get
is an unruby-esque method name in that in contains a verb and additionally puts the verb dangling at the end, if anything, it should read get_instance_variable.
Its weird name should remind us, DON'T DO THIS.
Similarly, in front end development, it is considered bad practice to use tags like <strong>
and <em>
in your HTML file; it's more proper to separate styling into a dedicated CSS file. It’s cleaner that way. Although <strong>
and <em>
aren’t technically deprecated, w3 schools put it this way:
“Tip: [The <strong>
] tag is not deprecated, but it is possible to achieve richer effect with CSS.”
In other words, these tags are HTML syntactic vinegar.
In conclusion, be appreciative of syntactic sugar in Ruby and in English. Here’s a life tip: You don’t need to spell out “et cetera.” None of us really know how to spell it without looking it up. Just say etc. and be grateful that it’s socially acceptable. Love, syntactic sugar.
Hello, world!!
It's been a long time coming, but I am finally learning to code!
I can remember the first time I felt the urge. The year was 2005 (or so). I figured out how to change the background and text colors of my MySpace page. Never had I felt more victorious.
But the internet was young, as was I, and I didn't feel compelled to build anything of my own. Those kinds of ideas just weren't brewing in me... yet. It wasn't until I hit the working world that I started to identify holes that I knew I could fill, if only I had the right tools.
In 2011, Amazon hired me to help launch a new flash-sale site, MyHabit, which would compete against online retailers like Gilt and RueLaLa. Although I was hired as a Buyer, I found myself obsessed with helping my team become more efficient in planning and building our sales, and I spend a disproportionate amount of time building Excel-based workflow tools. Although I loved working with product, I wanted to be optimizing workflows all the time. Which led me to Razorfish.
There, I worked on several web development projects as a Functional Analyst. It was my primary responsibility to identify business requirements and ensure that all requirements were accounted for in the final design. Effectively, I worked closely with UX and Visual Designers to deliver a functional product. Consequently, I also spent a lot of time doing QA before a product was delivered, and thereby worked hand-in-hand with developers to identify bugs and implement changes.
These experiences were wildly educational for me and opened my eyes to e-commerce, software, agency life, Agile methodology, and much more. Most of all, working alongside brilliantly smart humans showed me what passion looks like in practice. But for everything I learned at each of these jobs, all the while I was contributing to project teams: brainstorming, iterating, coordinating... something was missing. I wanted to be the builder. Not the hand holder, not the documenter, the builder! Which brought me to The Flatiron School.
What is Flatiron? It's a 12-week intensive web development bootcamp that will spit me out ready to code in a professional setting. It's a community of 29 other career-changers (and some recent college grads) who are driven to learn a new hard skill in an environment that encourages teamwork over competition. It's a place that believes that writing code is empowering and with enough dedication, you can do it. Yes, you! I'm only 2 weeks in, and I already feel wholeheartedly that it's changing my life.
So stick around if you want to hear my ramblings, breakthroughs, complaints, and general geekery. This is going to be FUN.